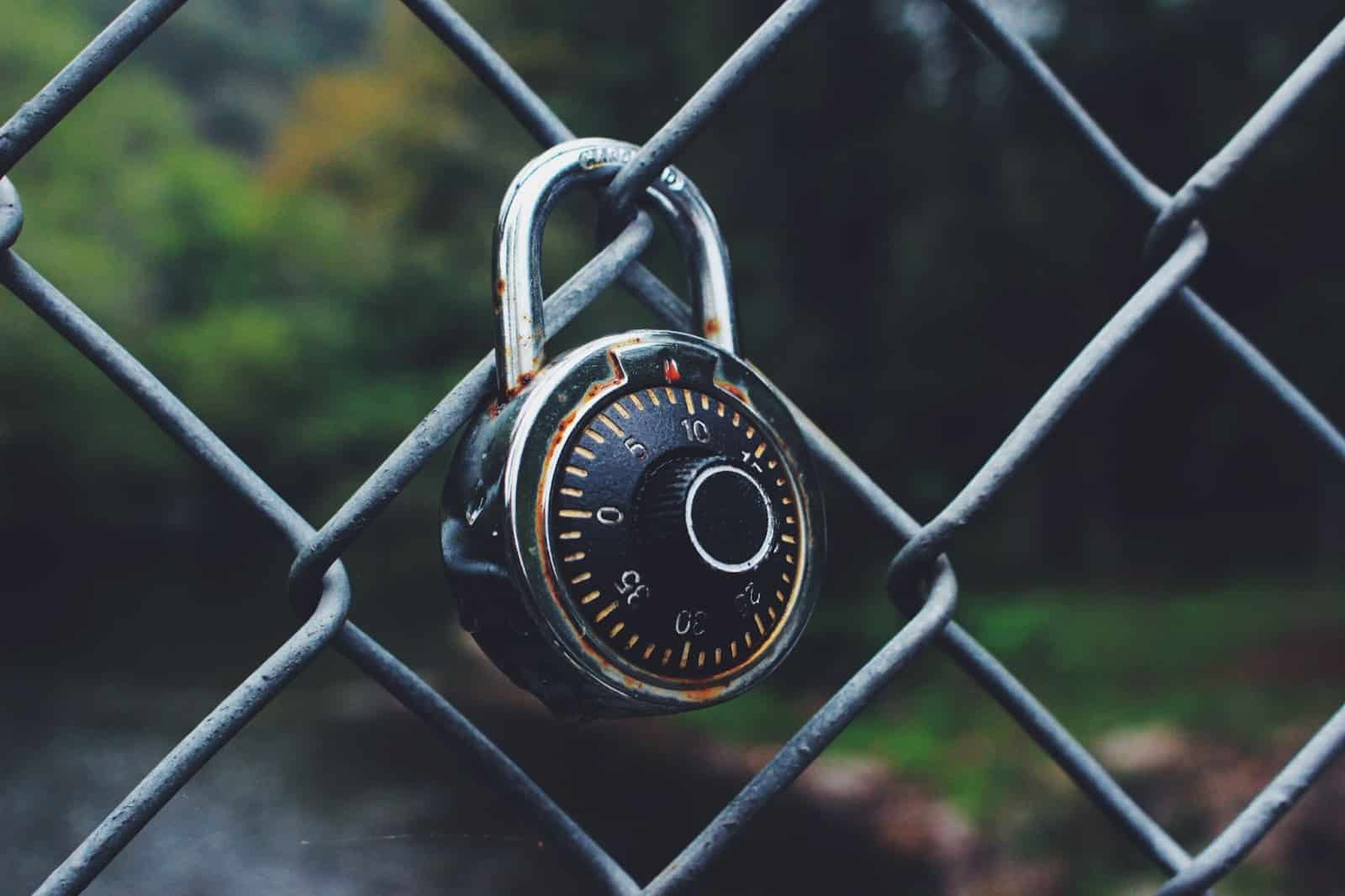
In this article, we’ll address broken authentication, explore the intricacies of authentication, and show you how to provide appropriate security mechanisms for your website.
First, I’ll briefly discuss what broken authentication is. Then I’ll use examples to illustrate what broken authentication attacks look like and which vulnerabilities they target. Finally, I’ll offer some mitigating strategies to address those vulnerabilities.
But before getting started, I want to clarify that this article is for .NET developers. With that out of the way, let’s jump right in.
What Is Broken Authentication?
“Broken authentication” is an umbrella term that describes several vulnerabilities that attackers can exploit to hijack your system and impersonate users.
An authentication mechanism ensures that only a verified user can access information and privileges on a web application. However, it’s “broken” when an attacker successfully bypasses the process and impersonates the user. Essentially, the attacker skips the login security and gains access to the privileges the hacked user has.
These vulnerabilities go hand-in-hand with poor session management and credential management. Attackers can disguise themselves as a user by hijacking a session ID or stealing login credentials. This kind of breach enables attackers to compromise passwords, keys or session tokens, user account information, and other details to assume user identities.
How Broken Authentication Happens
Let’s look at exactly how attackers can hijack your system.
Broken Authentication Through Poor Credential Management
Given a poor implementation of credential management, an attacker can hijack your authentication mechanism to gain access to the system.
There are various ways poor credential management allows attackers to achieve this. One is by allowing weak passwords like “12345” or “password.” When your system allows users to create weak passwords, an attacker can use password-cracking techniques like brute force, rainbow tables, and dictionaries.
Another way you might be allowing attackers to wreak havoc on your security is by using weak cryptography. When your system uses inadequate encryption mechanisms like base64 and hashing algorithms like SHA1 or MD5, your user credentials will be exposed during a breach.
Broken Authentication Through Poor Session Management
Whenever users interact with your website, your application issues session IDs and records their interactions. As stated by the OWASP broken authentication recommendations, this session ID is equivalent to your original login credentials. So, if an attacker were to steal your session ID, he can essentially impersonate your identity.
This kind of breach is called session hijacking.
Examples of Broken Authentication
Below are some examples of broken authentication attacks in detail.
Password Spraying
The term “password spraying” refers to the use of simple and weak passwords, such as “12345” or “password,” by attackers attempting to breach secure accounts.
Credential Stuffing
Whenever a breach happens on a system with a large user base with improperly encrypted credentials, users are vulnerable to credential stuffing.
Credential stuffing is the use of credentials obtained from other breaches. This approach assumes that a significant number of users will probably be using stolen credentials on different platforms.
Session Hijacking
You make your users susceptible to hijacking and impersonation when using session IDs. For example, suppose a user forgets to log off. In that case, any other person can hijack their session and act like the victim on your system. Additionally, if the same ID is issued before and after authentication, it could potentially open the door to an attack called session fixation.
Session ID URL
If your system implements session ID by appending it to the URL, any individual who can gain access to that URL can impersonate the user’s identity. Attackers can do this by hijacking insecure Wi-Fi connections, man in the middle attacks, or simple eavesdropping at the address bar.
Phishing Attacks
Finally, users can be susceptible to phishing attacks that send links to websites that look legitimate, convincing them to cede their login credentials. This last attack is quite notorious and challenging to address as it relies squarely on the user’s capacity to spot them.
Addressing Broken Authentication Vulnerabilities
In order to address the vulnerabilities above, you need to follow best practices from the Open Web Application Security Project, or OWASP. Thankfully, you can activate most of these recommendations in ASP.NET Core using the configuration file in your project.
Secure Password Storage
You must encrypt, hash, and salt all passwords. This protects users in case of a breach and slows down brute-force attacks and other malicious attempts. Thankfully, if you’re using the ASP.NET Core Identity framework, you already have secure password hashes and a unique salt mechanism implemented. ASP.NET Core Identity uses the PBKDF2 hashing function for passwords, and it generates a random salt per user.
Don’t Allow Weak Passwords
You must require all users to fulfill specific requirements when specifying their passwords. For example, setting passwords to a particular length and requiring special characters and letters as well as numbers helps prevent credential theft. Consequently, your system will reject passwords that don’t meet the requirements.
You can do this by modifying the IdentityOptions method like so:
services.Configure<IdentityOptions>(options => {
// Password settings
options.Password.RequireDigit = true;
options.Password.RequiredLength = 8;
options.Password.RequireNonAlphanumeric = true;
options.Password.RequireUppercase = true;
options.Password.RequireLowercase = true;
options.Password.RequiredUniqueChars = 6;
});
Keep in mind that some of these specifications protect users better than others, but they might also do the opposite if users won’t comply with them.
Add a Strict Credential Recovery Process
I recommend that you implement a strict process for credential recovery. This should involve several verification checks and steps that make it hard and costly for attackers to abuse.
You can achieve this by adding the following lines to the previous code:
services.Configure<IdentityOptions>(options => {
// Password settings
options.Password.RequireDigit = true;
options.Password.RequiredLength = 8;
options.Password.RequireNonAlphanumeric = true;
options.Password.RequireUppercase = true;
options.Password.RequireLowercase = true;
options.Password.RequiredUniqueChars = 6;
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(30);
options.Lockout.MaxFailedAccessAttempts = 10;
options.SignIn.RequireConfirmedEmail = true;
options.User.RequireUniqueEmail = true;
});
Implement Breached Password Protection
A breached password protection mechanism will lock the accounts of users whose passwords have been compromised. In addition, you can configure this mechanism to return access when users change their passwords. This way, you guarantee that the users have a chance to prevent damage if attackers steal their passwords.
You can implement this using a Git project called PwnedPassword that will provide an updated repository of breached passwords to cross-validate in real time.
Regulate Session Length
All web applications must end user sessions after a certain period of inactivity. The length of this period depends on the type of application. For example, a streaming platform might require a long period of inactivity, while a sensitive banking website should have a short time limit.
To regulate the length of a session, add the following lines to the project config file.
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddRazorPages();
builder.Services.AddControllersWithViews();
/// ADD THIS
builder.Services.AddDistributedMemoryCache();
/// ADD THIS
builder.Services.AddSession(options =>
{
options.IdleTimeout = TimeSpan.FromSeconds(10);
options.Cookie.HttpOnly = true;
options.Cookie.IsEssential = true;
});
var app = builder.Build();
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
/// ADD THIS
app.UseSession();
app.MapRazorPages();
app.MapDefaultControllerRoute();
app.Run();
Improve Session Management
It’s imperative that your platform provides individual session IDs after every successful authentication attempt. In addition, your system must invalidate old session IDs to prevent hijacking as soon as a session ends.
Don’t Use Session ID URLs
In all cases, you must secure web URLs with SSL without including the session ID.
Multifactor Authentication
Multifactor authentication (MFA) is one of the most robust protection mechanisms at your disposal. MFA requires an additional credential to verify a user’s identity. An example would be a one-time password (OTP) mailed or messaged to the user.
Phishing
It’s vital to provide regular education for users about the potential risks of phishing attacks and weak passwords. Sending monthly or quarterly emails reminding users to be vigilant and cautious will usually suffice.

Conclusion
It’s your job to implement robust and comprehensive security measures to ensure the safety of your assets and the data of your users. However, it’s challenging to keep up with the ever-evolving threats on the web. That’s why we recommend Dynamic Application Security Testing (DAST) from StackHawk. DAST runs security tests against a running application in real time, finding vulnerabilities your team introduced as well as exploitable open-source vulnerabilities like broken authentication.
You can read more about it and get started for free.
This post was written by Juan Reyes. Juan is an engineer by profession and a dreamer by heart who crossed the seas to reach Japan following the promise of opportunity and challenge. While trying to find himself and build a meaningful life in the east, Juan borrows wisdom from his experiences as an entrepreneur, artist, hustler, father figure, husband, and friend to start writing about passion, meaning, self-development, leadership, relationships, and mental health. His many years of struggle and self-discovery have inspired him and drive to embark on a journey for wisdom.