Implementing complex rules and mitigation policies for our platforms has become necessary in the last decades.
A lot has changed in terms of the intricacies of today's web. And, of course, we wouldn't dare to claim that 100% of the web is secure against the threats popping up daily.
Still, there is a significant movement from the security community to keep making security measures on the web more robust and effective against these threats. However, not all security measures are created equal. For example, one of the essential features to protect our users is HTTP Strict Transport Security, or HSTS.
The goal of this article is to explore the concepts of HSTS.
We'll briefly define what HSTS is and what makes it fundamental to secure communication between your server and the client, explore how to implement this security feature, and review some of the complications that might arise.
By the end, you should understand what HSTS is and how to incorporate it into your projects.
Note: This article is aimed at .NET developers, and thus, we will be exploring how to implement solutions to HSTS on the ASP.NET Core development stack. This means that to get the most out of this article, you need experience working with C# and ASP.NET.
If you're interested in the concepts of HSTS in general, we recommend you check our other articles on the topic focused on the technology stack of your preference.
With that out of the way, let's dive in.
Explaining HTTP Strict Transport Security
The following explanation will be technical and is relatively standard on all platforms, but bear with me.
Since its inception, encryption has been the first line of defense for protecting data from malicious users. Therefore, our job as developers is to ensure all transactions on our websites are encrypted with the proper protocols.
While the web encrypts and secures client-server transactions with SSL/TLS protocols, whether your transactions are protected is an entirely different matter.
Here's how the standard communication flow goes:
The communication flow between a website and a user first requires an HTTP request to the domain.
Then, if the server in question implements SSL/TLS with a valid certificate and enforces HTTPS rerouting, the user will be redirected (301) to the same site with an HTTPS request.
This flow ensures that all communication between the client and server is done securely through encryption.
Except, no, not really.
MITM Attack
We've discussed man-in-the-middle (MITM) attacks in previous posts, but here's a refresher.
A trusting user tries to access our website by reaching our server through a compromised network (a counterfeit access point concealed as legitimate).
In this case, the initial handshake can open the victim to vulnerabilities in the following way.
The attacker intercepts the communication between client and server, acting as the man in the middle.
He then rewrites all transactions between himself and the victim to be unencrypted.
Consequently, all transactions between the attacker and the server remain encrypted, misleading the server to believe the victim is protected.
Meanwhile, the attacker intersects all data the victim sends to our server.
Profit.
This attack is known as SSL stripping. It works by allowing the attacker to act as a communication intermediary. The attacker can then dictate the victim's security protocol, basically striping the client of any encryption security.
Not good.
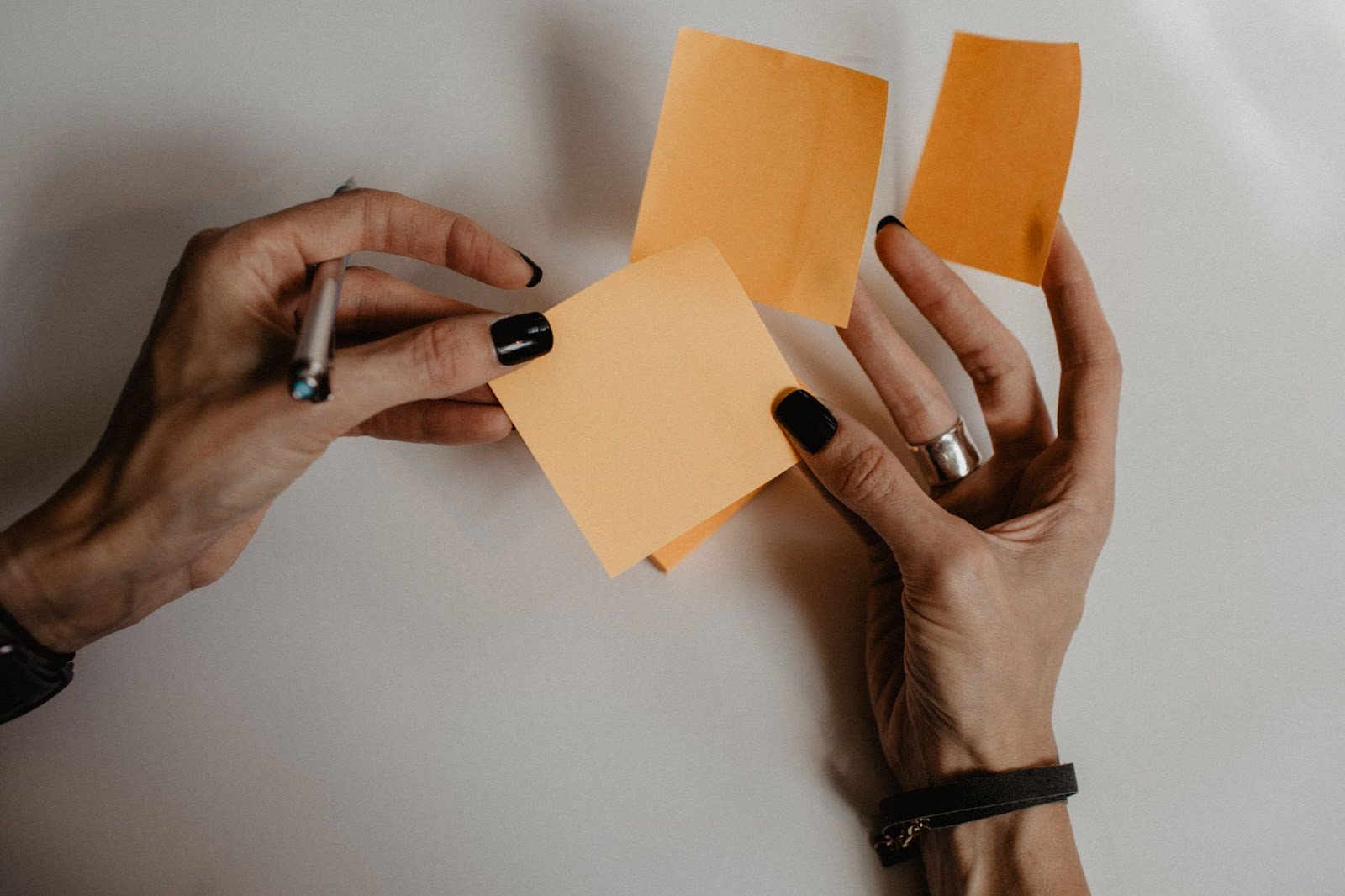
Our Options
As we can see, the most critical step in this attack is the initial handshake that the victim performs at the beginning. So, to prevent this exploit from occurring, we need to ensure that the users' browser communicates with our server using encryption exclusively. And the most effective way to do that is, well, to explicitly instruct the browser to do so.
This flow is, in essence, what HTTP Strict Transport Security represents, and it is one of the cornerstones of web security.
The Basics
Now that all the theory is out of the way, let's explore how we can secure our websites.
The good news is that, for the most part, our browsers' built-in security features get us most of the way there. All we need to do to implement the primary layer of security with HSTS is add the following header to your server responses.
Strict-Transport-Security: max-age=31536000; includeSubDomains; preload
HSTS headers contain three directives, one compulsory and two optional. Again, this should be familiar to you if you've read one of our previous posts on HSTS.
max-age: This states how long the browser will comply with the policy. Notice that we have set the value as 31536000, which equals one year. You can put any value you consider appropriate, but remember that your clients won't be able to access your site if there's an issue with your SSL certificate once the browser receives the HSTS policy.
includeSubDomains: This optional directive states whether the subdomains will need to comply with the policy. If you have mywebsite.com with an SSL certificate and set the header for clients who visit it, then www.mywebsite.com and subdomain.mywebsite.com will also be required to follow the same HSTS policy.
preload: This optional directive states that you want to add your site to the HSTS preload list included in the browser. This list essentially cements your website to follow HSTS for that client permanently.
Securing Your ASP.NET Core Project
You have two options for adding the HSTS header to an ASP.NET core project:
Implement HTTPS Redirection Middleware (UseHttpsRedirection) to redirect HTTP requests to HTTPS.
Implement HSTS Middleware (UseHsts) to send clients HTTP Strict Transport Security Protocol (HSTS) headers.
To use the UseHttpsRedirection method, modify your Program.cs file with the following:
app.UseHttpsRedirection();
Additionally, we need to set a port for the middleware to redirect an insecure request to HTTPS.
If no port is available, the page won't redirect to HTTPS, and the middleware logs the warning "Failed to determine the https port for redirect."
By adding the following directive to the appsettings.json file, you can easily do that.
{
"https_port": 443,
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*"
}
For the second route, you can add the HSTS middleware with the following code in the Program.cs file.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Error");
app.UseHsts();
}
builder.Services.AddHsts(options =>
{
options.Preload = true;
options.IncludeSubDomains = true;
options.MaxAge = TimeSpan.FromDays(60);
options.ExcludedHosts.Add("example.com");
options.ExcludedHosts.Add("www.example.com");
});
Of course, just because you know how to apply an HSTS header to a page doesn't mean your website can enforce the policy. For example, if your website isn't compatible with HSTS, you'll get this message:
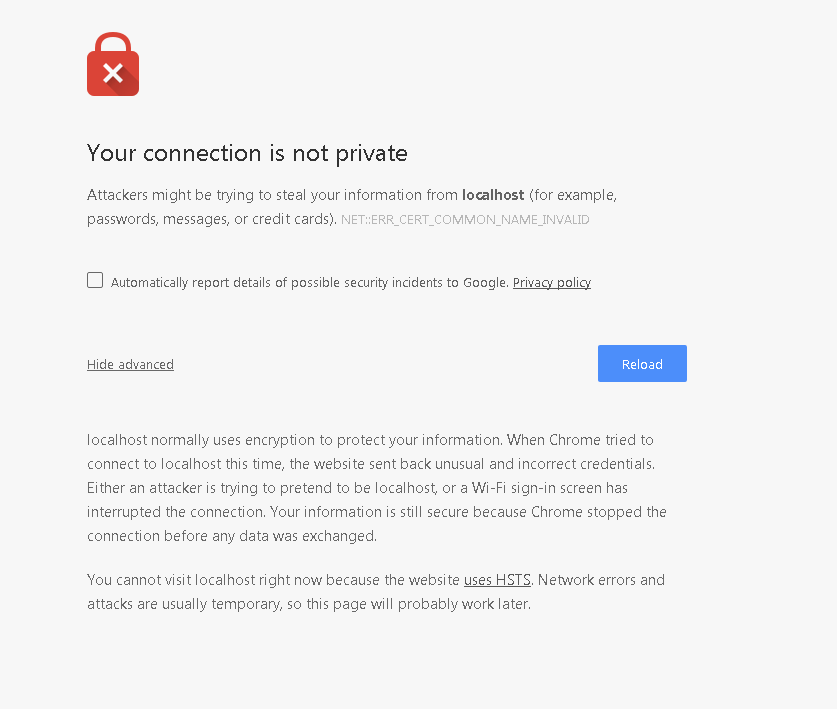
As we can see, an error prevents our browser from displaying the page.
Although the server we attempted to access indicates that the communication is encrypted, our browser received some suspicious information and cut the connection.

Understanding HSTS Errors
Once we see the error message the browser is providing us, we can have an idea of the possible origins of the problem.
The suspicious activity could be from an attacker trying to impersonate the server.
A WiFi login screen could be interfering with the loading process.
The server doesn't have the correct SSL/TLS configuration.
Our first course of action should be to ensure that we set up encryption correctly. Also, if we are deploying HSTS for the first time, we must have a proper implementation plan.
Taking some notes from Scott Helme's HSTS tutorial, here's a checklist of steps to follow.
Find all subdomains that belong to your site (consult DNS CNAME entries). Note that they might belong to third-party services.
Confirm that the root domain and its subdomains are accessible via HTTPS.
Make sure you configured proper redirection of HTTP to HTTPS.
Set a short expiration time. For example, max-age=300 (5 minutes).
Append the includeSubDomains directive if necessary.
Increment max-age in stages. Strive for two years of validity.
Once all is good, add the preload directive.
Submit your domain to Google's HSTS preload list, which will ensure that future versions of all major browsers have your domain preloaded and marked as secure-only. (Optional)
After following all these steps, you will have a site enforcing HTTPS communication only. From that point on, all users will obey the policy.
Final Thoughts on HSTS
In our quest to implement a reliable security layer for our applications, a lot has to be done to provide the necessary assurance to build a robust level of trust with our users.
Today's web has pushed the envelope of security and standards quite far, making some solutions complex and challenging.
Thankfully, implementing SSL/TLS and HSTS policy is quite simple and goes a long way to protect a large portion of the web.
This post was written by Juan Reyes. Juan is an engineer by profession and a dreamer by heart who crossed the seas to reach Japan following the promise of opportunity and challenge. While trying to find himself and build a meaningful life in the east, Juan borrows wisdom from his experiences as an entrepreneur, artist, hustler, father figure, husband, and friend to start writing about passion, meaning, self-development, leadership, relationships, and mental health. His many years of struggle and self-discovery have inspired him and drive to embark on a journey for wisdom.