In this article, we'll discuss broken authentication in Vue and how to address it effectively in your projects.
We'll start by defining what broken authentication is. Then we'll explore the two common methods that facilitate broken authentication vulnerabilities. Next, we'll show you some examples of the most common broken authentication vulnerabilities on the web. Finally, we'll offer effective mitigation practices.
By the end of this article, you can expect to understand the fundamentals of authentication, how broken authentication affects your security, and what you can do about it.
We wrote this article for developers with experience in Vue and JavaScript. However, if this is not your development stack of choice, we encourage you to find an article with your technology of choice on our blog.
Defining Broken Authentication
To explain what broken authentication is, let's briefly revisit the definition shared in a related article. As previously stated, "The term broken authentication is an umbrella used for several vulnerabilities that attackers can exploit to hijack our systems and impersonate other users."
Authentication is the mechanism in your platform that ensures that only a verified user can access the information and privileges provided on a web application.
We refer to authentication as broken when a bad actor successfully circumvents the procedure, whether by impersonating a user or acquiring privileges.
Going back to the referenced article: "To be more specific, broken authentication refers to the vulnerabilities or weaknesses inherent in an online platform or application's authentication mechanisms. We can usually find these vulnerabilities in poor session management and credential management." Both of these avenues could lead to broken authentication since bad actors can impersonate users, either by hijacking the session ID or stealing the login credentials of other users.
Furthermore, this breach allows bad actors to compromise passwords, session tokens, user information, and other elements to take over user identities.
Broken Authentication Methods
Now that you know that broken authentication attacks target credential and session management mechanisms, let's see how bad actors can seize these systems.
Deficient Credential Management
If your system incorrectly implements credential management, a bad actor can hijack your authentication mechanism and gain access to the system. One of the ways this happens is when you allow weak passwords.
When your system allows users to use passwords like '12345' or 'password,' a bad actor can make use of tools and techniques like brute force, rainbow tables, and dictionaries to crack them.
The second way bad actors can take over your security is by exploiting weak cryptography. Inadequate encryption mechanisms like base64 and hashing algorithms like SHA1 or MD5 puts user credentials in a vulnerable spot in the case of a security breach.
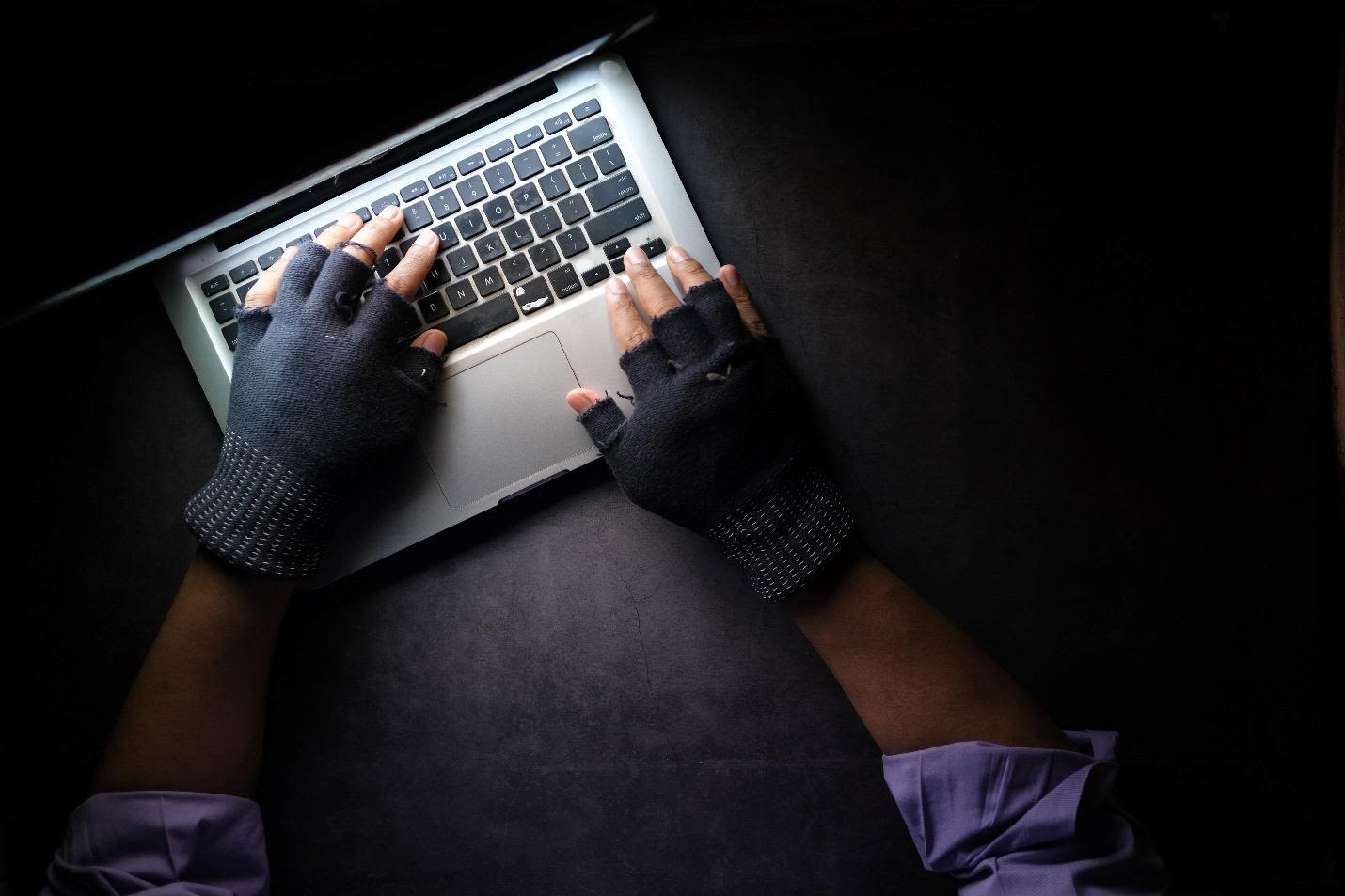
Inadequate Session Management
As stated by the OWASP, the typical interaction flow between a server and client starts with issuing a session ID and registering the interaction. This session ID is analogous to login credentials. Therefore, if a bad actor successfully steals this session ID, he can impersonate the victim. This is known as a session hijacking breach, and it's common on the web.
Broken Authentication Vulnerabilities
There are many ways to take advantage of poor implementation of session and credential management systems. Here are a few of them.
Password Spraying
Password spraying exploits accounts that use simple and weak passwords such as '12345' and 'password,' rainbow tables, and password dictionaries.
Credential Stuffing
Credential stuffing takes advantage of credentials obtained from previous breaches from other platforms. This approach presumes that many users reuse credentials due to laziness or friction between platforms.
Session Hijacking
When you expose the user session IDs, you make your users susceptible to hijacks by bad actors. One example is when a user forgets to log off before leaving and a bad actor hijacks the session to act like the victim. Furthermore, when your system issues the same session ID before and after authentication, it could open the door to a session fixation attack.
Session ID URL
Following the previous point, if you implement session ID by adding it to the URL as a query string, any individual gaining access to that URL can impersonate the victim. Bad actors can achieve this by hijacking insecure Wi-Fi connections, man-in-the-middle attacks, or simply looking at the victim's address bar.
Phishing Attacks
By sending emails disguised as legitimate, bad actors can make victims click on links to websites that appear legitimate, persuading them to disclose their credentials.
Fixing Broken Authentication
The best way to mitigate these vulnerabilities requires following the best practices laid out by the OWASP. However, this can be a complicated and tricky process that can unintentionally break some things.
The good news is that you can address most of the issues by integrating robust, third-party solutions like Auth0. Their platform will protect your users' credentials and provide you with the best tools to secure your application.
Let's briefly see how to implement it.
Integrating Third-Party Authentication
The first thing you need to do is create an account on the Auth0 website. Once you've done that, create your application and get the keys necessary to integrate the library here.
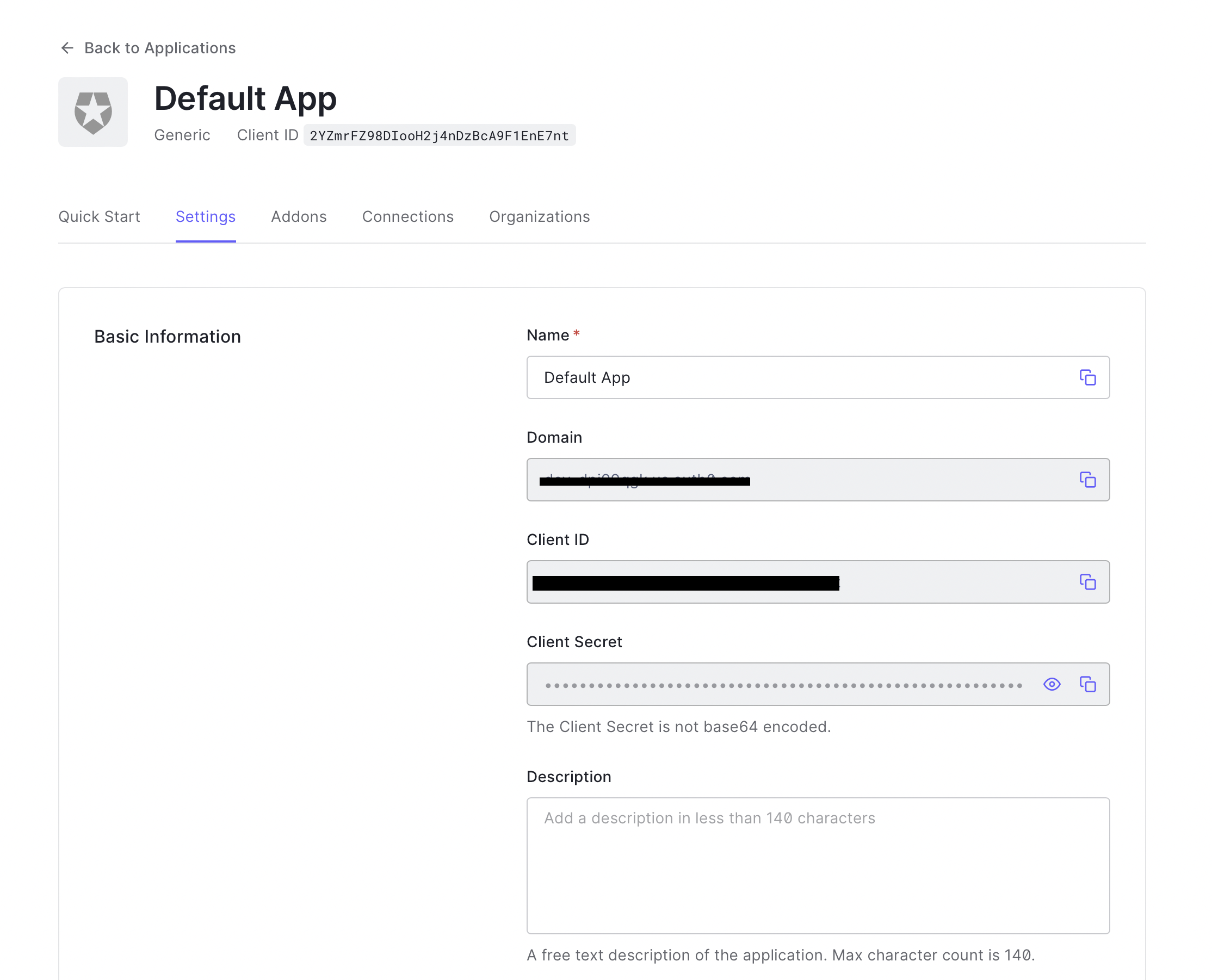
All you need to do is provide the callback and logout URLs, which should point to your application. Once you do that, make sure to specify your domain in the Allowed Web Origins.
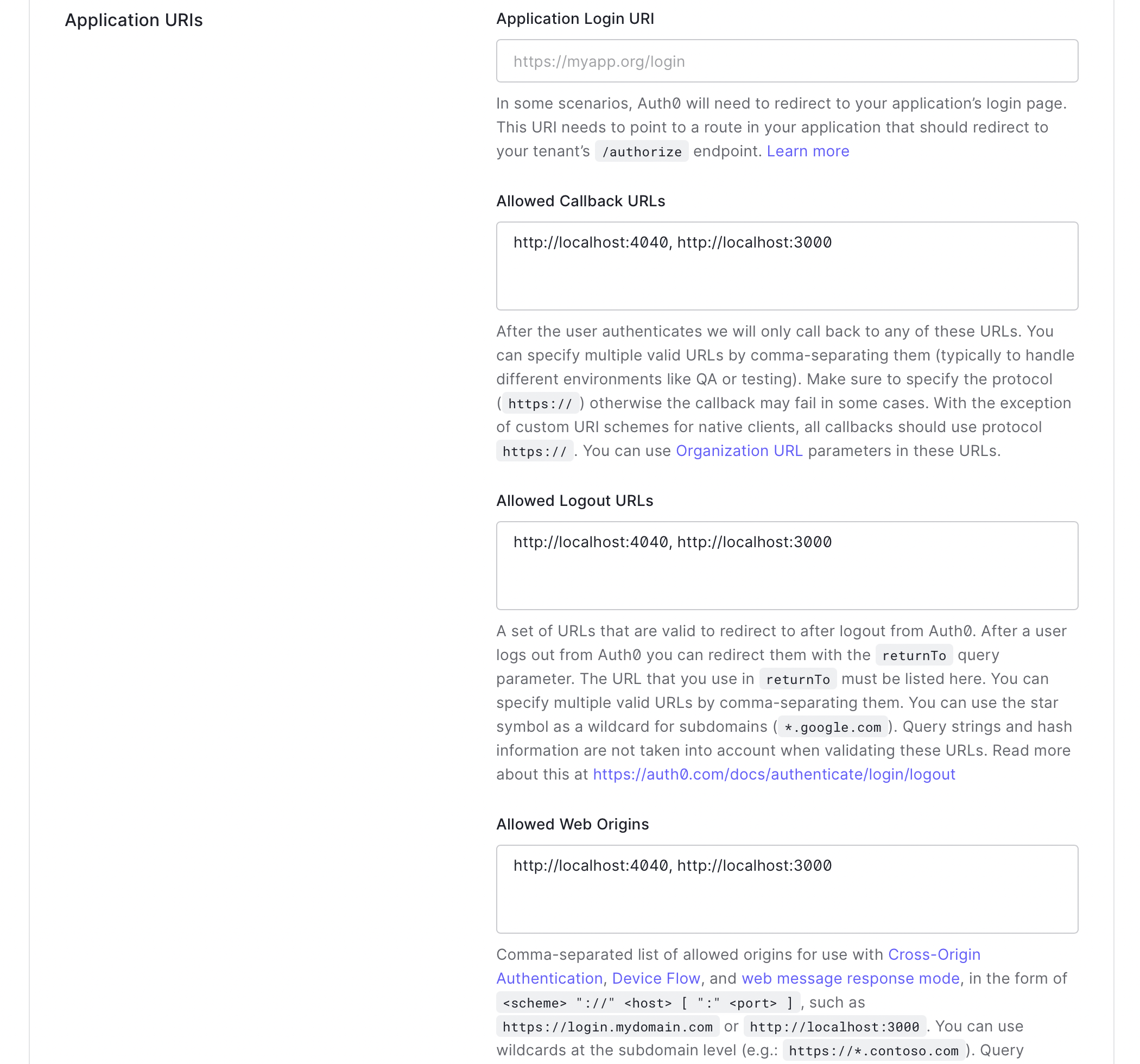
Finally, save the ClientID and Secret provided by the application in a safe place. These will be the credentials with which your application will communicate and interact with Auth0.
Now, proceed to your application console and type the following command:
npm install @auth0/auth0-vue
Then in your main app class file, add the following code, replacing the placeholders with your credentials.
import { createAuth0 } from '@auth0/auth0-vue';
const app = createApp(App);
app.use(
createAuth0({
domain: "YOUR_DOMAIN",
client_id: "YOUR_CLIENT_ID",
redirect_uri: window.location.origin
})
);
app.mount('#app');
Finally, add the proper login code to make use of the Auth0 library. As stated in the official Auth0 documentation for Vue: "To add a login to your application, use the 'loginWithRedirect'
function that is exposed on the return value of 'useAuth0'
, which you can access in your component's setup function."
<template>
<div>
<button @click="login">Log in</button>
</div>
</template>
<script>
import { useAuth0 } from '@auth0/auth0-vue';
export default {
setup() {
const { loginWithRedirect } = useAuth0();
return {
login: () => {
loginWithRedirect();
}
};
}
};
</script>
This code is a simple example of making use of the login feature. You can use it as the basis of your specific implementation that satisfies your needs.
Please check the official documentation if you want to learn more about integrating Auth0 into Vue.
Beyond this, make sure to follow the best practices and guidelines to minimize the potential for exploitation. Among the most critical steps are the following:
Secure password storage
It is essential that you encrypt, hash, and salt all passwords in the database. This ensures that users' credentials are safeguarded in case of a breach.
Thankfully, Auth0 takes care of this for you.
Don't allow weak passwords
Weak passwords must be heavily discouraged and, if possible, prevented. In addition, users must be required to meet specific requirements when creating their passwords, like password length and the inclusion of special characters.
You can do a lot of this on the front end by specifying restrictions on the user forms. However, if your users use external authentication providers like Google or Facebook, they have their own password policies.
Add a strict credential recovery process
We recommend that you implement a mechanism for credential recovery. This should require some verification steps that make it cumbersome for bad actors to abuse.
Control session length
The best course of action against session shenanigans is to end the user sessions after a period of inactivity.
You can change this in the Auth0 dashboard by going to Settings, then Advanced, and then scrolling to the login session management segment. More info here.
Improve session management
Your platform must provide unique session IDs after every authentication process. Additionally, you must invalidate old session IDs as soon as a session ends.
Disregard session ID URL
The information on web URLs must be protected with SSL and must not include session data.
Phishing
The best defense against phishing is teaching your users to identify and disregard phishing attempts. Additionally, share your communication policies so they know what to expect from you.

Conclusion
Finally, a lot more can be done to reduce attacks from bad actors. Of course, there's always somewhere else to put effort, from implementing sophisticated biometric or two-factor authentication mechanisms to running regular penetration tests to strengthen your system.
In this case, we recommend you consider our solution at StackHawk. Our dynamic application security testing (DAST) solution for your team is a great and robust solution that will empower your team's capacity to protect your assets and clients.
Check it out here.
This post was written by Juan Reyes. Juan is an engineer by profession and a dreamer by heart who crossed the seas to reach Japan following the promise of opportunity and challenge. While trying to find himself and build a meaningful life in the east, Juan borrows wisdom from his experiences as an entrepreneur, artist, hustler, father figure, husband, and friend to start writing about passion, meaning, self-development, leadership, relationships, and mental health. His many years of struggle and self-discovery have inspired him and drive to embark on a journey for wisdom.